Devices must be authenticate before start to send data. Easyberry Iot has a JWT (Token) authentication available. Let do how it works.
In this post, we are going to support us in POSTMAN software. You can download it if you wish or use our Easyberry LAB section to make your first tests.
TOKEN Authentication
With token-type authentication, user submission and password are not required with each data submission, thereby reducing the exposure of our credentials. This process requires two steps.
- Token Request
- Sending Data
NOTE: once the token is obtained, it is not required to send the user and pass again.
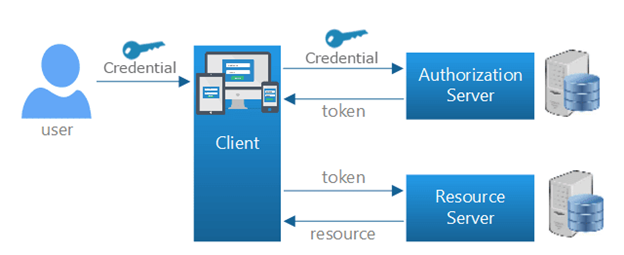
With Jquery
Step 1:
Token request
var username = “user”;
var password = “pwd”;
var url = ‘mysite.com’;
$ .ajax ({
url: url,
success: function (json) {
alert (“Success”, json);
},
error: function (XMLHttpRequest, textStatus, errorThrown) {
alert (textStatus, errorThrown);
},
type: ‘POST’,
contentType: ‘json’,
data: {username: <user>, password: <password>},
});
This request returns the token that must be stored in the RAM of the devices or if you want to save it in non-volatile memory make sure you encrypt those to not compromise your app.
Step 2:
Sending data
var url = ‘mysite.com’;
$ .ajax ({
url: url,
success: function (json) {
alert (“Success”, json);
},
error: function (XMLHttpRequest, textStatus, errorThrown) {
alert (textStatus, errorThrown);
},
// headers: {‘Authorization’: ‘Basic bWFkaHNvbWUxMjM =’},
beforeSend: function (xhr) {
xhr.setRequestHeader (“Authorization”, “Bearer” + <token>);
},
type: ‘POST’,
contentType: ‘json’,
data: <JSON string>
});
Example using POSTMAN
Token Request:
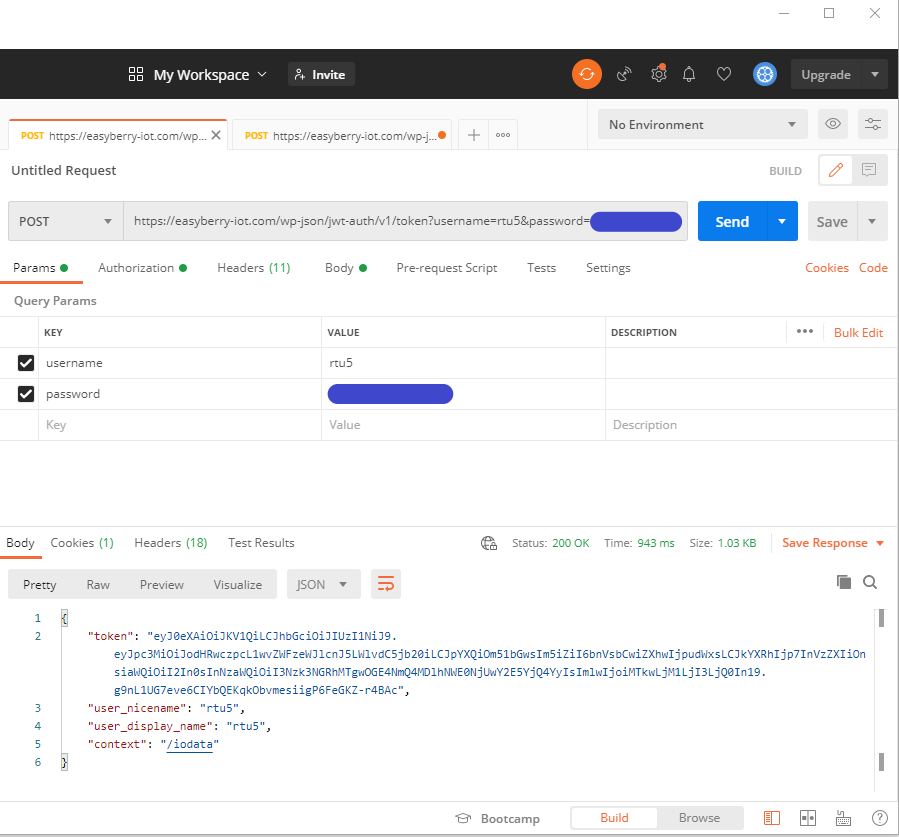
Sending Data:
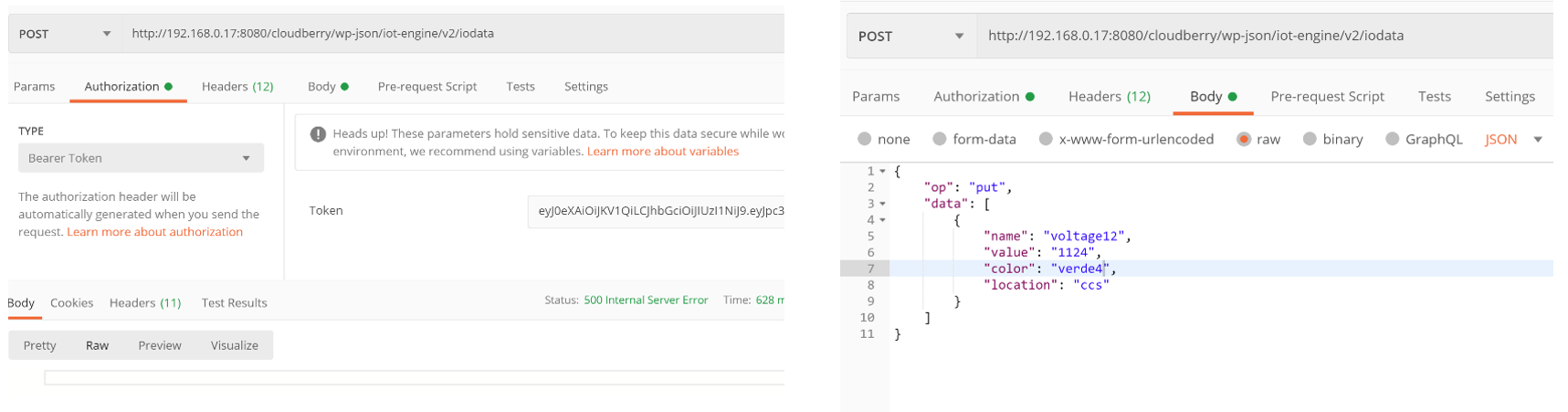
There are two processes by which we can request and send data. And they are GET and PUT respectively through the formats and / or models established in JSON to communicate with the Easyberry IoT data engine (API REST).
Sending data to Easyberry IoT
https://easyberry-iot.com/wp-json/iot-engine/v2/iodata
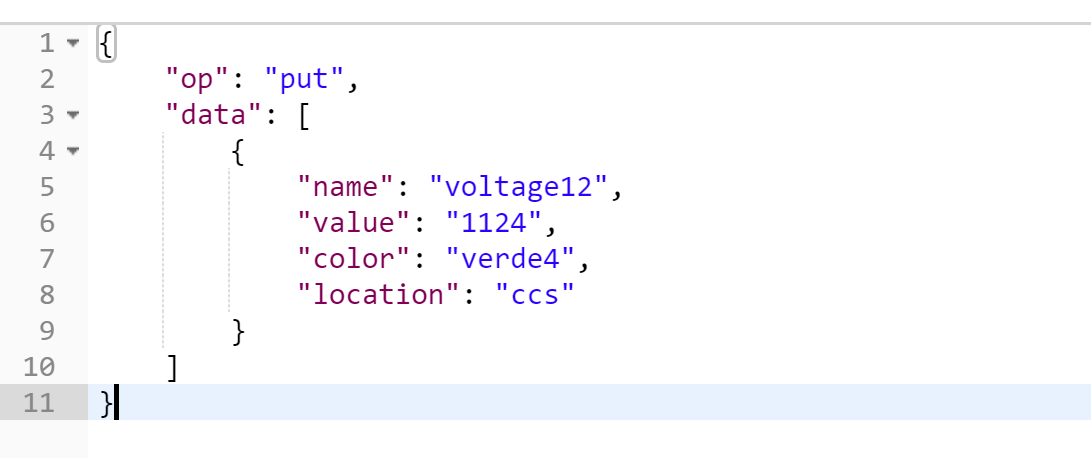
Data request to Easyberry. You can make requests for a single object or “thing” or you can do it using grouped objects “context” or “dataset”. For more information see how a context and / or datasets are defined here.
Data Objects Summary and examples
GET Functions
- {“op”:”get”,”thing”:[“thing1″,”thing2”,…]}
- {“op”:”get”,”context”:”[“context1″,”context”,…]}
- {“op”:”get”,”dataset”:[“datasetname,…”]}
- {“op”:”get”,”list”:”[things|contexts|datasets]”}
- {“op”:”get”,”list”:”things”}
- {“op”:”get”,”list”:”contexts”}
- {“op”:”get”,”list”:”datasets”}
PUT Functions
- Put things:
{“op”:”put”,
“data”:[{“name”:”register name”,
“value”:”register value”,
“epoch”:”timestamp(optional)”,
“properties”:{“name property 1″:”property value 1”,
“name property 2″:”property value 2”,
}
},
{“name”:”register name 2″,
“value”:”register value 2″,
“epoch”:”timestamp(optional)”,
“properties”:{“name property 1″:”property value 1”,
“name property 2″:”property value 2”
}
}
]
- Put datasets:
{“op”:”put”,”dataset”:[….]} available soon…
0 Comments